🖇️ 문제 링크
코딩테스트 연습 - 기둥과 보 설치
빙하가 깨지면서 스노우타운에 떠내려 온 "죠르디"는 인생 2막을 위해 주택 건축사업에 뛰어들기로 결심하였습니다. "죠르디"는 기둥과 보를 이용하여 벽면 구조물을 자동으로 세우는 로봇을 개발할 계획인데, 그에 앞서 로봇의 동작을 시뮬레이션 할 수 있는 프로그램을 만들고 있습니다. 프로그램은 2차원 가상 벽면에 기둥과 보를 이용한 구조물을 설치할 수 있는데, 기둥과 보는 길이가 1인 선분 으로 표현되며 다음과 같은 규칙을 가지고 있습니다.

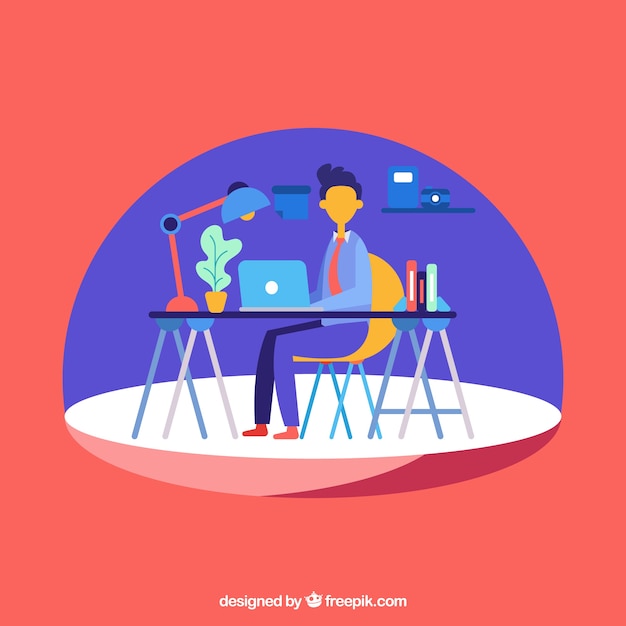
📝 문제 분석
기둥 → 바닥 위에 있거나 보의 한쪽 끝 부분 위에 있거나, 또는 다른 기둥 위에 있어야 함
보 → 한쪽 끝 부분이 기둥 위에 있거나, 또는 양쪽 끝 부분이 다른 보와 동시에 연결되어 있어야 함
리스트에 기둥과 보를 순서대로 입력합니다. 만약 기둥이나 보를 넣거나 삭제했을 때, 위의 조건에 부합하지 않는다면 다시 복원합니다.
⌨️ 코드
import java.util.*;
class Solution {
ArrayList<Build> ret = new ArrayList<>();
public int[][] solution(int n, int[][] build_frame) {
for (int[] arr : build_frame) {
Build s = get(arr[0], arr[1], arr[2]);
// 설치
if (arr[3] == 1) {
ret.add(s);
// 설치 안되면 다시 지움
if (check())
ret.remove(s);
} else {
ret.remove(s);
// 삭제 안되면 다시 넣음
if (check())
ret.add(s);
}
}
// 출력 형태 맞추기
int[][] answer = new int[ret.size()][3];
int i = 0;
Collections.sort(ret);
for(Build b : ret)
answer[i++] = new int[]{b.x, b.y, b.a};
return answer;
}
boolean check() {
for(Build b : ret) {
int x = b.x, y = b.y, a = b.a;
// 기둥일 때
// 밑에 바닥이거나, 기둥이거나, 보의 끝일 때
if(a == 0) {
if(y != 0 &&
!ret.contains(get(x, y - 1, 0)) &&
!ret.contains(get(x - 1, y, 1)) &&
!ret.contains(get(x, y, 1))) return true;
} else {
// 한 쪽이 기둥이거나, 양쪽 끝이 보일 때
if(!ret.contains(get(x, y - 1, 0)) &&
!ret.contains(get(x + 1, y - 1, 0)) &&
!(ret.contains(get(x - 1, y, 1)) && ret.contains(get(x + 1, y, 1))))
return true;
}
}
return false;
}
Build get(int x, int y, int a) {
return new Build(x, y, a);
}
static class Build implements Comparable<Build>{
int x, y, a;
Build(int x, int y, int a) {
this.x = x;
this.y = y;
this.a = a;
}
@Override
public int compareTo(Build o) {
if(this.x != o.x) return this.x - o.x;
if(this.y != o.y) return this.y - o.y;
return this.a - o.a;
}
@Override
public boolean equals(Object o) {
Build build = (Build) o;
return x == build.x && y == build.y && a == build.a;
}
}
}
Uploaded by Notion2Tistory v1.1.0